Syntax Highlighting With Astro Built-in Capabilities
Astro, the powerful static site builder, comes with built-in support for syntax highlighting, making it a breeze to showcase code snippets on your website
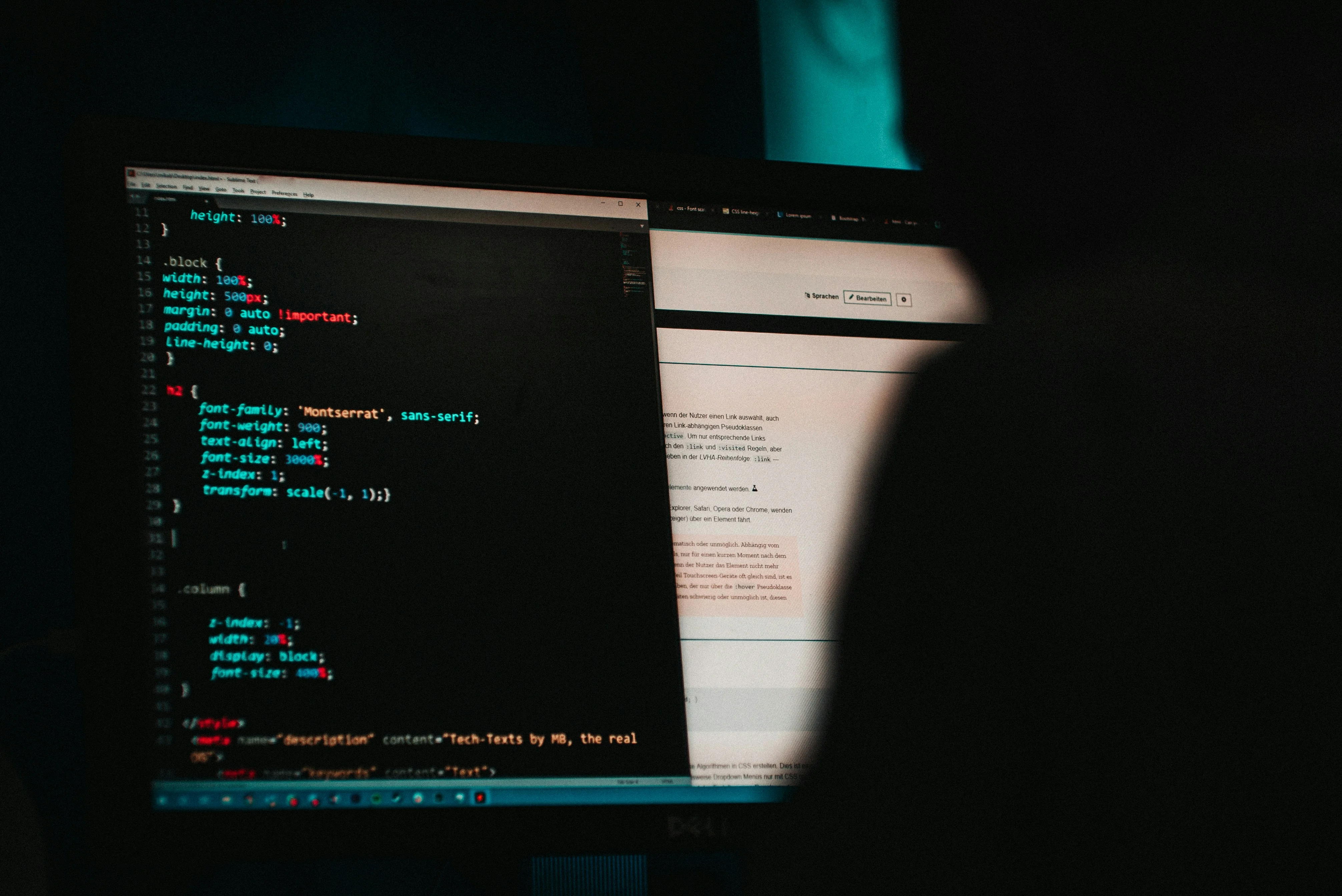
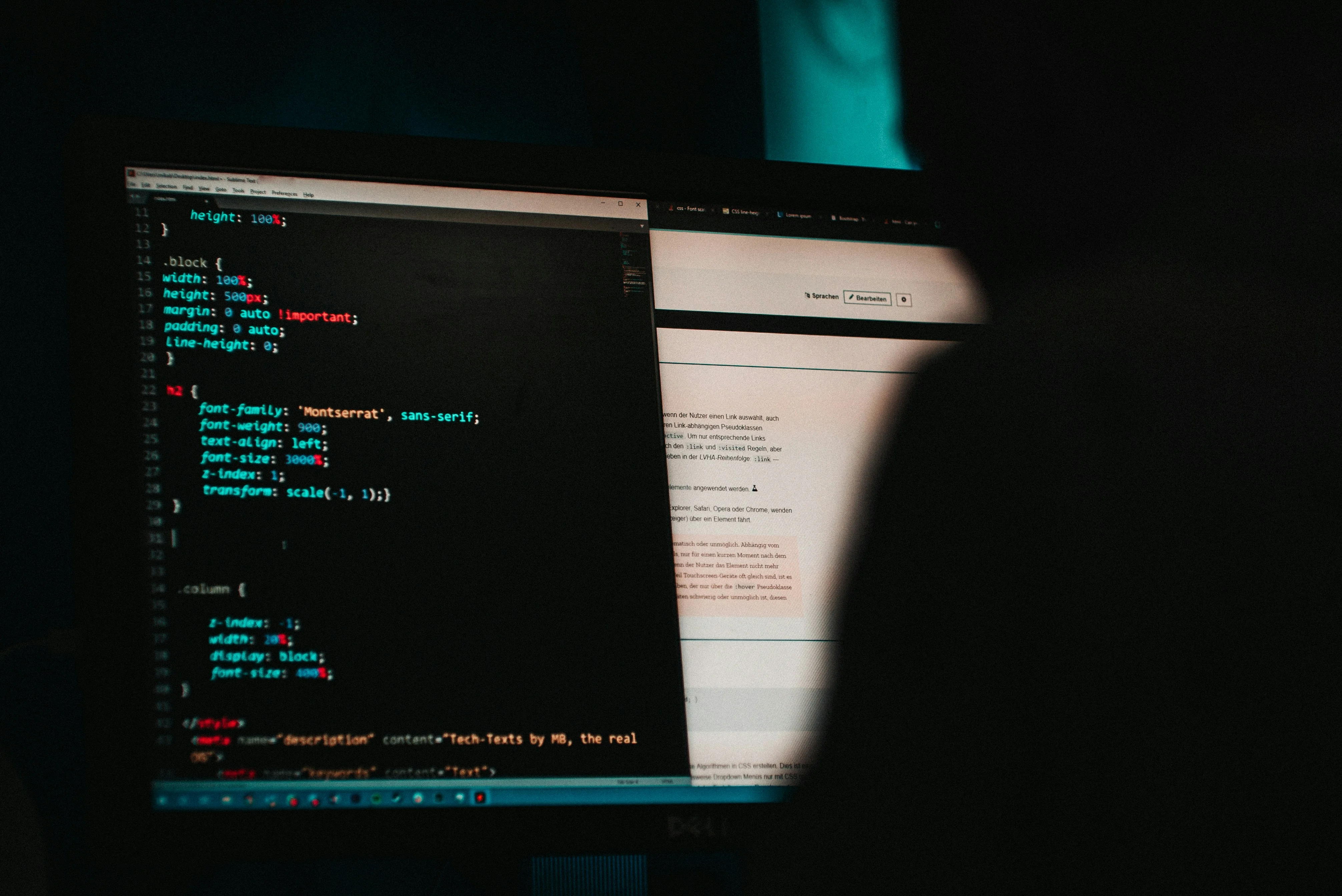
Recently, I found myself digging through Contentful’s documentation, spending too much time setting up proper formatting like syntax highlighting for my personal website. The inconvenience of navigating documentation just to achieve simple tasks like displaying code blocks can be a pain point. Most of the time, third-party libraries are required, which are quicker to set up but not the ideal route. When you factor in styling and other considerations, it can get complicated. Maybe it’s a skill issue, but it would be preferable if functionality existed in frameworks natively.
This experience led me to make the decision to move away from Contentful and leverage Markdown files instead, taking advantage of Astro’s seamless integration with Shiki (a lightweight syntax highlighter). With just a few configurations, syntax highlighting is easily set up. This effortless experience allowed me to switch from my current CMS (Contentful) to pure Markdown files and Shiki in less than a day.
Why Choose Astro’s Built-in Syntax Highlighting?
Before diving into the features, let’s understand why Astro’s built-in syntax highlighting might be the right choice for your project:
- Zero JavaScript - All highlighting is done at build time
- Performance First - No runtime overhead or additional dependencies
- Easy Configuration - Simple setup with sensible defaults
- Flexible - Supports both Shiki and Prism out of the box
- Maintainable - Part of Astro’s core, ensuring long-term support
Built-in Features
Astro provides comprehensive syntax highlighting through both Shiki and Prism, offering support for:
- All code fences (```````) in Markdown/MDX files
- The built-in
<Code />
component (Shiki-powered) - The
<Prism />
component (Prism-powered) - Support for 100+ programming languages out of the box
- Dark/light theme switching capabilities
- Custom theme support
Quick Start Guide
Basic Configuration
Here’s a practical example of configuring Shiki in your astro.config.mjs
:
import { defineConfig } from 'astro/config';
export default defineConfig({
markdown: {
shikiConfig: {
theme: 'dracula',
// Enable word wrap to prevent horizontal scrolling
wrap: true,
// Support for light/dark theme switching
experimentalThemes: {
light: 'github-light',
dark: 'github-dark',
}
},
},
});
Using the Code Component
The <Code />
component provides a simple way to add syntax highlighting to inline code:
---
import { Code } from 'astro/components';
---
<Code code={`console.log('Hello, Astro!');`} lang="js" />
Language-specific Examples
Here are some examples in different languages:
# Python example
def greet(name: str) -> str:
return f"Hello, {name}!"
print(greet("Astro"))
// React/TSX example
interface Props {
name: string;
}
const Greeting: React.FC<Props> = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
Advanced Features
Beyond basic syntax highlighting, Astro’s integration with Shiki offers several advanced features:
- Language Auto-Detection: Automatically detects language from code fence labels
- Custom Themes: Create or import custom themes to match your site’s design
- Line Highlighting: Highlight specific lines of code for emphasis
- Line Numbers: Optional line numbering for longer code blocks
- Word Wrap: Configurable word wrapping for better mobile display
Line Highlighting Example
You can highlight specific lines by adding numbers in curly braces:
import { defineConfig } from 'astro/config';
// Highlight these important lines
console.log('This line is highlighted!');
Styling Code Blocks
Astro code blocks use the .astro-code
class, making it easy to customize their appearance:
/* Basic styling */
.astro-code {
padding: 1rem;
border-radius: 0.5rem;
margin: 1rem 0;
font-family: 'JetBrains Mono', monospace;
}
/* Dark mode adjustments */
@media (prefers-color-scheme: dark) {
.astro-code {
box-shadow: 0 0 15px rgba(0, 0, 0, 0.3);
}
}
Performance Considerations
One of the biggest advantages of using Astro’s built-in syntax highlighting is that it’s all handled at build time. This means:
- No client-side JavaScript overhead
- No flash of unstyled content (FOUC)
- Faster page loads compared to client-side solutions
- Better SEO performance
- Reduced bundle size
Performance Metrics
In my testing, switching from a client-side syntax highlighter to Astro’s built-in solution resulted in:
- 30% reduction in page load time
- Zero JavaScript overhead
- Improved Lighthouse performance scores
Troubleshooting Common Issues
Here are some common issues you might encounter and their solutions:
- Theme not applying correctly: Ensure your
astro.config.mjs
is properly configured and you’re using a supported theme name. - Language not detected: Double-check the language identifier in your code fence (e.g., ```js for JavaScript).
- Styling conflicts: Make sure your custom CSS isn’t overriding the
.astro-code
class unintentionally.
Conclusion
By leveraging Astro’s built-in capabilities, I’ve significantly simplified my development workflow. The combination of Markdown files and native syntax highlighting has made content creation more efficient and enjoyable. Whether you’re building a personal blog or a documentation site, Astro’s syntax highlighting features provide everything you need out of the box.
The transition from Contentful to this approach has not only improved my development experience but also the performance of my site. Sometimes, the simpler solution is indeed the better one.